Crafting a Custom Linux Kernel for Your Embedded Projects
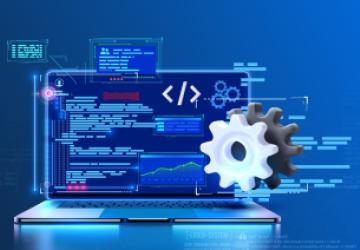
Introduction
Embedded systems have become a cornerstone of modern technology, powering everything from IoT devices to automotive control systems. These specialized systems rely on software that is lightweight, efficient, and highly optimized for specific hardware configurations. At the heart of this software stack lies the Linux kernel, which is widely used in embedded devices due to its flexibility, robustness, and open-source nature.
However, the generic Linux kernel is often bloated with unnecessary drivers, modules, and features that are irrelevant for embedded applications. For developers working on embedded systems, building a custom Linux kernel is not only a means to optimize performance but also a way to reduce the system's resource consumption, improve security, and enhance hardware compatibility.
In this article, we will guide you through the intricate process of building a custom Linux kernel for embedded systems. We will explore the reasons for kernel customization, the necessary prerequisites, step-by-step instructions for configuring, compiling, and deploying the kernel, and finally, best practices to ensure stability and performance in production environments.
Why Customize the Linux Kernel for Embedded Systems?
Performance OptimizationOne of the key reasons to build a custom Linux kernel for an embedded system is performance. The default kernel comes packed with features that are designed to work across a wide range of hardware platforms, but these general-purpose features are often unnecessary in embedded applications. By removing unused drivers and modules, you can significantly improve system performance, reduce boot times, and optimize resource usage. This allows the embedded system to run faster, with fewer interruptions and lower power consumption, which is crucial for devices with limited computational power or battery life.
For instance, an IoT device running on an ARM-based processor doesn't need support for high-performance networking protocols or advanced graphical interfaces. Customizing the kernel ensures that only the essential features are included, thus reducing overhead.
Reduced Resource ConsumptionEmbedded systems often operate with limited memory, storage, and CPU power. A lean, stripped-down kernel can minimize memory usage, helping the device operate more efficiently. By eliminating unnecessary features such as unused file systems, debugging symbols, and kernel-level services, you can conserve valuable system resources. This is especially important for real-time embedded systems, where even small inefficiencies can result in delayed responses or missed deadlines.
Enhanced SecurityA custom Linux kernel also enhances security by reducing the attack surface. Embedded systems are frequently deployed in environments where security is critical, such as automotive systems or medical devices. By disabling vulnerable or unnecessary kernel features, you can prevent potential exploits. Additionally, you can implement kernel security mechanisms like SELinux, AppArmor, or Seccomp to further harden the system against malicious attacks.
Improved Boot TimesIn many embedded applications, fast boot times are a key requirement. Removing unnecessary drivers and modules from the kernel can lead to much faster initialization, reducing the time it takes for the device to reach an operational state. For example, devices used in industrial automation or automotive systems may need to boot in a matter of seconds. A custom kernel tailored for quick startup is essential in such cases.
Hardware CompatibilityMany embedded systems rely on specialized hardware components that require specific kernel configurations. A custom kernel allows you to ensure full compatibility with the embedded hardware, such as the System on Chip (SoC), peripherals, and sensors. This also means you can add support for new or proprietary hardware not included in the standard kernel distribution.
Prerequisites for Building a Custom Kernel
Before diving into the actual process of building a custom Linux kernel, certain prerequisites must be met. These involve both hardware and software requirements.
Hardware RequirementsTo begin, you need the target embedded system’s hardware or a development board that mimics the actual production environment. Common platforms for embedded systems include:
- ARM-based boards like Raspberry Pi, BeagleBone, or STM32.
- SoCs like Qualcomm Snapdragon or NXP i.MX.
- Embedded x86 or MIPS architectures.
In addition to the hardware, you may need peripheral components such as network interfaces, serial consoles, or storage devices for testing the kernel.
Software RequirementsBuilding a custom kernel requires a Linux-based development environment. Ubuntu, Debian, or other Linux distributions are commonly used for this purpose. The following essential software packages are needed:
- Kernel Source Code: You can download the kernel source from the Linux Kernel Archives.
- Cross-compilation Toolchain: For embedded systems, cross-compilation is necessary to compile the kernel for a different architecture (e.g., ARM) than the one running on your development machine.
- GNU Make and GCC: These are the tools used to compile the kernel.
Install these tools on your Linux development environment with the following command:
sudo apt-get install build-essential libncurses-dev bc git flex bison
To set up the development environment for cross-compilation, download and install a cross-compiler specific to your target architecture. For example, for ARM-based systems:
sudo apt-get install gcc-arm-linux-gnueabi
This cross-compiler allows you to build a kernel on your development machine that can run on an ARM-based embedded system.
Obtaining and Configuring the Linux Kernel
Downloading the Kernel SourceThe first step in building a custom kernel is downloading the source code. This can be done by cloning the kernel repository or downloading a stable release from the Linux Kernel Archives:
wget https://cdn.kernel.org/pub/linux/kernel/v5.x/linux-5.10.77.tar.xz tar -xf linux-5.10.77.tar.xz cd linux-5.10.77
Ensure that the kernel version you download is compatible with your embedded hardware. Some embedded platforms may have specific kernel versions that work best with their hardware components.
Kernel Configuration OptionsOnce the kernel source is downloaded, the next step is to configure it. This involves enabling or disabling specific features, drivers, and modules based on the needs of your embedded system. You can use the following command to launch the kernel configuration interface:
make menuconfig
The menuconfig
interface provides a menu-based system to configure the kernel. Focus on the following critical areas:
- Processor Type and Features: Set the architecture to match your embedded device (e.g., ARM, x86, MIPS).
- Device Drivers: Enable only the necessary drivers for your embedded hardware. Disable drivers for components that won’t be used, such as sound cards or graphics interfaces if they are irrelevant to your device.
- File Systems: If your embedded system uses a specific file system (e.g., ext4 or JFFS2), enable support for it while disabling others.
For example, if your embedded system uses ARM, navigate to "Processor Type and Features" and select ARM-specific options:
Processor Type and Features -> ARM system type -> Select your platform
Carefully consider the specific hardware and software requirements of your embedded system, and tweak the configuration accordingly.
Cross-Compiling the Linux Kernel
Why Cross-Compiling is NecessaryEmbedded systems often run on architectures that differ from the one used in development. Cross-compiling allows developers to build a kernel on their desktop (e.g., x86 architecture) and produce a binary that will run on the embedded device (e.g., ARM architecture).
Setting Up the Cross-CompilerEnsure that the appropriate cross-compiler is installed. For an ARM-based embedded system, you will need the ARM cross-compiler toolchain:
sudo apt-get install gcc-arm-linux-gnueabihf
For MIPS systems, a MIPS cross-compiler is necessary:
sudo apt-get install gcc-mips-linux-gnu
To build the kernel using the cross-compiler, use the following commands. First, clean any old build files:
make ARCH=arm CROSS_COMPILE=arm-linux-gnueabi- clean
Next, compile the kernel with the cross-compiler:
make ARCH=arm CROSS_COMPILE=arm-linux-gnueabi- -j$(nproc)
Here, -j$(nproc)
allows the build process to use all available CPU cores on your development machine to speed up the compilation.
If the build is successful, the compiled kernel image will be available in the arch/arm/boot/
directory. For ARM systems, the image is typically named zImage
or Image
.
Installing and Testing the Custom Kernel
Copying the Kernel to the Embedded DeviceOnce the kernel is compiled, the next step is to transfer it to the embedded system. This can be done using various methods:
- SD Card or USB: If the embedded device boots from an SD card or USB, you can copy the kernel image directly to the boot partition.
- Network Transfer: For systems connected over the network, tools like SCP can be used to transfer the kernel image:
scp arch/arm/boot/zImage user@embedded-device:/boot/
To boot the embedded system with the new kernel, the bootloader (such as U-Boot or GRUB) must be configured to load the new kernel image. For U-Boot, update the boot configuration:
setenv bootcmd 'fatload mmc 0:1 0x80000000 zImage; bootz 0x80000000' saveenv
Reboot the device to test the new kernel.
Troubleshooting Boot or Kernel IssuesIf the device fails to boot or encounters kernel panics, it is important to debug the issue using serial console logs. Connect to the serial port of the device and use a terminal application like minicom
or screen
to capture boot logs. Look for error messages that indicate missing drivers or hardware support, and adjust the kernel configuration accordingly.
Optimizing the Custom Kernel for Production
Reducing Kernel SizeFor embedded systems with limited storage, reducing the size of the kernel is crucial. Techniques for minimizing kernel size include:
- Disabling Debugging Features: Disable kernel debugging symbols by configuring
CONFIG_DEBUG_KERNEL=n
in the kernel configuration. - Using Compression: Compress the kernel image using gzip or LZMA to further reduce its size:
make ARCH=arm CROSS_COMPILE=arm-linux-gnueabi- zImage.gz
In production environments, security is paramount. Enable security modules such as SELinux or AppArmor during kernel configuration. Additionally, apply kernel security patches that address known vulnerabilities and use kernel features like Seccomp to limit the system calls available to untrusted processes.
Testing for Stability and PerformanceBefore deploying the custom kernel in production, thoroughly test it for stability. Use tools like stress-ng
or sysbench
to perform stress tests, ensuring the system can handle maximum load without crashing. Verify that all hardware components are functioning correctly, and monitor system logs for any unusual behavior.
Kernel updates are a necessary part of maintaining a secure and stable embedded system. When applying updates, ensure that the changes do not introduce new bugs or performance regressions. It is a good practice to use version control (e.g., Git) to track changes to the kernel configuration and source code.
Best Practices and Common Pitfalls
Kernel Customization TipsWhen customizing the kernel, it is advisable to make incremental changes and test frequently. Start by disabling only a few features at a time, and verify that the system still functions as expected. This reduces the likelihood of introducing hard-to-debug issues.
Common Errors and FixesCompilation errors are common when building a custom kernel. Some tips for resolving them include:
- Missing Headers: Ensure all necessary development packages are installed.
- Driver Issues: Double-check the kernel configuration to ensure that required drivers are enabled.
Document every change made to the kernel configuration, including which features were enabled or disabled and why. Use Git to manage kernel versions and track changes over time, allowing you to revert to a previous stable version if necessary.
Conclusion
Building a custom Linux kernel for embedded systems is a powerful way to optimize performance, enhance security, and tailor the system to specific hardware requirements. By carefully selecting kernel features and drivers, you can significantly improve the efficiency of the embedded device, reduce resource consumption, and meet strict production requirements.
While the process may seem complex, the rewards of a finely-tuned kernel are well worth the effort. Whether you're working on a small IoT device or a mission-critical automotive system, mastering kernel customization will give you greater control over your embedded systems and help you build more reliable, secure, and performant products.