How to Divide Two Variables in Bash Scripting
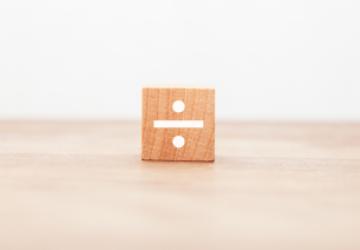
Introduction
Bash scripting is a powerful tool for automating tasks on Linux and Unix-like systems. While it's well-known for managing file and process operations, arithmetic operations, such as division, play a crucial role in many scripts. Understanding how to correctly divide two variables can help in resource allocation, data processing, and more. This article delves into the nuances of performing division in Bash, providing you with the knowledge to execute arithmetic operations smoothly and efficiently.
Basic Concepts
Understanding Variables in BashIn Bash, a variable is a name assigned to a piece of data that can be changed during the script execution. Variables are typically used to store numbers, strings, or file names, which can be manipulated to perform various operations.
Overview of Arithmetic OperationsBash supports basic arithmetic operations directly or through external utilities. These operations include addition, subtraction, multiplication, and division. However, Bash inherently performs integer arithmetic, which means it can only handle whole numbers without decimals unless additional tools are used.
Introduction to Arithmetic CommandsThere are two primary ways to perform arithmetic operations in Bash:
expr
: An external utility that evaluates expressions, including arithmetic calculations.- Arithmetic expansion
$(( ))
: A feature of Bash that allows for arithmetic operations directly within the script.
Setting Up Your Script
Creating a Bash Script FileTo start scripting, create a new file with a .sh
extension using a text editor, such as Nano or Vim. For example:
nano myscript.sh
After writing your script, you need to make it executable with the chmod
command:
chmod +x myscript.sh
A Bash script typically starts with a shebang (#!
) followed by the path to the Bash interpreter:
#!/bin/bash # Your script starts here
Declaring Variables
Assigning ValuesTo declare and assign values to variables in Bash, use the following syntax:
var1=10 var2=5
These variables can now be used in arithmetic operations.
Performing Division
Usingexpr
The expr
command is useful for integer division:
result=$(expr $var1 / $var2) echo "The result is $result"
This will output the result of dividing var1
by var2
.
Handling Integer Division
Since expr
only supports integer arithmetic, dividing two integers that do not divide evenly will result in the truncation of the decimal.
$(( ))
Arithmetic expansion allows for more straightforward syntax and direct script integration:
result=$(($var1 / $var2)) echo "The result is $result"
This method is cleaner and does not spawn an external process, making it faster than expr
.
Dealing with Non-Integer Results
Challenges of Floating-Point DivisionBash does not natively support floating-point arithmetic, which can complicate divisions that result in non-integer values.
Workarounds for Floating-Point DivisionTo handle floating-point division, you can use the bc
tool, an arbitrary precision calculator language:
result=$(echo "$var1 / $var2" | bc -l) echo "The result is $result"
This command sends the division operation to bc
, which handles the floating-point arithmetic and returns the result.
Common Pitfalls and Errors
Division by ZeroAttempting to divide by zero will cause an error in your script. It's important to check that the denominator is not zero before performing division:
if [ $var2 -eq 0 ]; then echo "Error: Division by zero." else result=$(($var1 / $var2)) echo "The result is $result" fi
Ensure that the inputs are numeric to avoid runtime errors:
if ! [[ "$var1" =~ ^[0-9]+$ ]] || ! [[ "$var2" =~ ^[0-9]+$ ]]; then echo "Error: Non-numeric input." else result=$(($var1 / $var2)) echo "The result is $result" fi
Practical Examples
Interactive Script for User InputCreating a script that takes user inputs and performs division:
#!/bin/bash echo "Enter two numbers: " read var1 var2 if [[ "$var2" -eq 0 ]]; then echo "Cannot divide by zero." else result=$(echo "$var1 / $var2" | bc -l) echo "Division result: $result" fi
Conclusion
This article has covered the essentials of performing division in Bash scripting, from integer operations to handling floating-point numbers. By understanding these principles, you can enhance your scripts' capabilities and perform complex calculations with ease. Experiment with these techniques to refine your scripting skills and tackle more advanced problems.